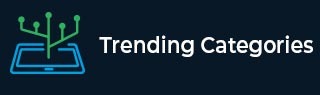
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find minimum value to insert at beginning for all positive prefix sums in Python
Suppose we have a list of numbers called nums. We have to find the minimum positive value that we can insert at the beginning of nums so that that prefix sums of the resulting list contains numbers that are all larger than 0.
So, if the input is like nums = [3, -6, 4, 3], then the output will be 4, because if we insert 4 to the list then we have [4, 3, -6, 4, 3]. Now the prefix sums are then [4, 7, 1, 5, 8], all are larger than 0.
To solve this, we will follow these steps −
insert 0 into nums at position 0
for i in range 1 to size of nums - 1, do
nums[i] := nums[i] + nums[i - 1]
return 1 - minimum of nums
Example
Let us see the following implementation to get better understanding
def solve(nums): nums.insert(0, 0) for i in range(1, len(nums)): nums[i] += nums[i - 1] return 1 - min(nums) nums = [3, -6, 4, 3] print(solve(nums))
Input
[3, -6, 4, 3]
Output
4
Advertisements