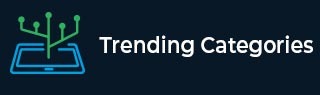
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find minimum number of days to eat N oranges in Python
Suppose we have a number n. So consider there are n oranges in the kitchen and we eat some of these oranges every day maintaining these rules: 1. Eat single orange. 2. If n is even, then eat n/2 oranges. 3. If n is divisible by 3 can eat 2*(n/3) oranges. We can select only one option each day. We have to find the minimum number of days to eat n oranges.
So, if the input is like n = 10, then the output will be 4 because
On day 1 eat 1 orange, 10 - 1 = 9.
On day 2 eat 6 oranges, 9 - 2*(9/3) = 9 - 6 = 3.
On day 3 eat 2 oranges, 3 - 2*(3/3) = 3 - 2 = 1.
On day 4 eat the last orange 1 - 1 = 0.
To solve this, we will follow these steps −
Define a function fun() . This will take n
if n is in memo, then
return memo[n]
if n<=2, then
return n
memo[n]:= 1 + minimum of (n mod 2+ fun(quotient of n/2)) and (n mod 3 + fun(quotient of n/3))
return memo[n]
From the main method, do the following
memo:= a new map
return fun(n)
Example
Let us see the following implementation to get better understanding
def solve(n): def fun(n): if n in memo: return memo[n] if n<=2: return n memo[n]=1+min(n%2+fun(n//2),n%3+fun(n//3)) return memo[n] memo={} return fun(n) n = 12 print(solve(n))
Input
7, [5,1,4,3]
Output
4