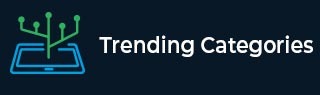
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find maximum value by inserting operators in between numbers in Python
Suppose we have a list of numbers called nums, we have to find the maximal value that can be generated by adding any binary operators like +, −, and * between the given numbers as well as insert any valid brackets.
So, if the input is like nums = [−6, −4, −10], then the output will be 100, as we can make the expression like: ((−6) + (−4)) * −10.
To solve this, we will follow these steps −
OPS := a list of operators [+, −, *]
N := size of A
if all elements in A is 0, then
return 0
Define a function dp() . This will take i, j
if i is same as j, then
return a pair (A[i], A[i])
low := inf, high := −inf
for k in range i to j − 1, do
for each left in dp(i, k), do
for each right in dp(k + 1, j), do
for each operator op in OPS, do
res := left op right
if res < low, then
low := res
if res > high, then
high := res
return pair (low, high)
From the main method do the following −
ans := dp(0, N − 1)
return second value of ans
Let us see the following implementation to get better understanding −
Example
import operator class Solution: def solve(self, A): OPS = [operator.add, operator.sub, operator.mul] N = len(A) if not any(A): return 0 def dp(i, j): if i == j: return [A[i], A[i]] low = float("inf") high = float("−inf") for k in range(i, j): for left in dp(i, k): for right in dp(k + 1, j): for op in OPS: res = op(left, right) if res < low: low = res if res > high: high = res return [low, high] return dp(0, N − 1)[1] ob = Solution() nums = [−6, −4, −10] print(ob.solve(nums))
Input
[−6, −4, −10]
Output
100