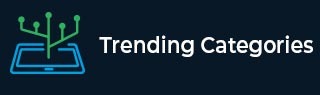
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find maximum number of consecutive values you can make in Python
Suppose we have an array called coins with n elements, and it is representing the coins that we own. The value of the ith coin is denoted as coins[i]. We can make some value x if we can select some of our n coins such that their values sum up to x. We have to find the maximum number of consecutive values that we can get with the coins starting from and including 0.
So, if the input is like coins = [1,1,3,4], then the output will be 10, because
0 = []
1 = [1]
2 = [1,1]
3 = [3]
4 = [4]
5 = [4,1]
6 = [4,1,1]
7 = [4,3]
8 = [4,3,1]
9 = [4,3,1,1]
To solve this, we will follow these steps −
sort the list coins
ans := 1
for each coin in coins, do
if coin > ans, then
come out from the loop
ans := ans + coin
return ans
Example
Let us see the following implementation to get better understanding −
def solve(coins): coins.sort() ans = 1 for coin in coins: if coin > ans: break ans+=coin return ans coins = [1,1,3,4] print(solve(coins))
Input
[1,1,3,4]
Output
10