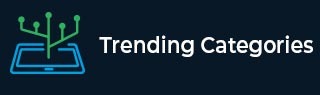
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find maximum average pass ratio in Python
Suppose we have a list of classes where classes[i] represents [pass_i, total_i] represents the number of students passed the examination of ith class and total number of students of the ith class respectively. We also have another value extra. This indicates extra number of brilliant students that are guaranteed to pass the exam of any class they are assigned to. We have to assign each of the extra students to a class in a way that maximizes the average number of passed student across all the classes. The pass ratio of a class determined by the number of students of the class that will passed divided by the total number of students of the class. And the average pass ratio is the sum of pass ratios of all the classes divided by the number of the classes. We have to find the maximum possible average pass ratio after assigning the extra students.
So, if the input is like classes = [[2,3],[4,6],[3,3]], extra = 3, then the output will be 0.83809, because two extra students at first class and add one extra student to second class to maximize the ratio, so now average is (4/5 + 5/7 + 3/3)/3 = 0.83809.
To solve this, we will follow these steps −
h := a list of tuples like (a/b-(a + 1)/(b + 1), a, b) for each pair (a, b) in classes
heapify h
while extra is non-zero, do
(v, a, b) := top of h, and delete it from h
(a, b) := (a + 1, b + 1)
insert (-(a + 1) /(b + 1) + a / b, a, b) into heap
extra := extra - 1
return average from all tuples of h
Example
Let us see the following implementation to get better understanding −
import heapq def solve(classes, extra): h = [(a / b - (a + 1) / (b + 1), a, b) for a, b in classes] heapq.heapify(h) while extra: v, a, b = heapq.heappop(h) a, b = a + 1, b + 1 heapq.heappush(h, (-(a + 1) / (b + 1) + a / b, a, b)) extra -= 1 return sum(a / b for v, a, b in h) / len(h) classes = [[2,3],[4,6],[3,3]] extra = 3 print(solve(classes, extra))
Input
[[2,3],[4,6],[3,3]], 3
Output
0