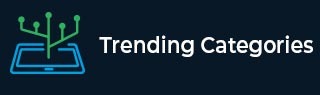
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find lexicographically largest mountain list in Python
Suppose we have three positive numbers say n, lower, and upper. We have to find a list whose length is n and that is strictly increasing and then strictly decreasing and all the numbers are in range [lower and upper] (both inclusive). And each increasing and decreasing parts should be non-empty. We have to find the lexicographically largest such list possible, if this is not possible, then return empty list.
So, if the input is like n = 5 lower = 3 upper = 7, then the output will be [6, 7, 6, 5, 4], if we look closely, the [7, 6, 5, 4, 3] is not valid because the strictly increasing part should be non-empty.
To solve this, we will follow these steps −
if n > 2 * (upper - lower) + 1, then
return empty list
c := upper - lower
d := 1
if c < n, then
d := n - c - 1
if d is same as 0, then
d := 1
f := a new list from range from (upper - d) to (upper - 1)
g := a new list from range (upper - n + d - 1) down to upper
concatenate f and g and return
Example
Let us see the following implementation to get better understanding
def solve(n, lower, upper): if n > 2 * (upper - lower) + 1: return [] c = upper - lower d = 1 if c < n: d = n - c - 1 if d == 0: d = 1 f = list(range(upper - d, upper)) g = list(range(upper, upper - n + d, -1)) return f + g n = 5 lower = 3 upper = 7 print(solve(n, lower, upper))
Input
5, 3, 7
Output
[6, 7, 6, 5, 4]