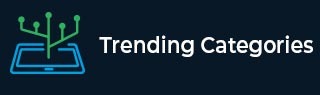
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find length of longest substring with character count of at least k in Python
Suppose we have a string s where each characters are sorted and we also have a number k, we have to find the length of the longest substring such that every character occurs at least k times.
So, if the input is like s = "aabccddeeffghij" k = 2, then the output will be 8, as the longest substring here is "ccddeeff" here every character occurs at least 2 times.
To solve this, we will follow these steps −
- Define a function rc() . This will take lst
- c := a map with all characters and their occurrences
- acc := a new list
- ans := 0
- valid := True
- for each x in lst, do
- if c[x] < k, then
- valid := False
- ans := maximum of ans and rc(acc)
- acc := a new list
- otherwise,
- insert x at the end of acc
- if c[x] < k, then
- if valid true, then
- return size of acc
- otherwise,
- ans := maximum of ans and rc(acc)
- return ans
- From the main method, do the following −
- return rc(a new list by converting each character of s)
Let us see the following implementation to get better understanding −
Example
from collections import Counter class Solution: def solve(self, s, k): def rc(lst): c = Counter(lst) acc = [] ans = 0 valid = True for x in lst: if c[x] < k: valid = False ans = max(ans, rc(acc)) acc = [] else: acc.append(x) if valid: return len(acc) else: ans = max(ans, rc(acc)) return ans return rc(list(s)) ob = Solution() s = "aabccddeeffghij" k = 2 print(ob.solve(s, k))
Input
"aabccddeeffghij", 2
Output
8
Advertisements