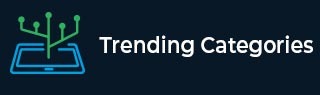
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find length of longest sublist containing repeated numbers by k operations in Python
Suppose we have a list called nums and a value k, now let us consider an operation by which we can update the value of any number in the list. We have to find the length of the longest sublist which contains repeated numbers after performing at most k operations.
So, if the input is like nums = [8, 6, 6, 4, 3, 6, 6] k = 2, then the output will be 6, because we can change 4 and 3 to 6, to make this array [8, 6, 6, 6, 6, 6, 6], and the length of sublist with all 6s is 6.
To solve this, we will follow these steps −
if nums is empty, then
return 0
num_count := an empty map
max_count := 0
start := 0
for each index end and value num in nums, do
num_count[num] := num_count[num] + 1
max_count := maximum of max_count and num_count[num]
if end - start + 1 > max_count + k, then
num_count[nums[start]] := num_count[nums[start]] - 1
start := start + 1
return end - start + 1
Example
Let us see the following implementation to get better understanding
from collections import defaultdict def solve(nums, k): if not nums: return 0 num_count = defaultdict(int) max_count = 0 start = 0 for end, num in enumerate(nums): num_count[num] += 1 max_count = max(max_count, num_count[num]) if end - start + 1 > max_count + k: num_count[nums[start]] -= 1 start += 1 return end - start + 1 nums = [8, 6, 6, 4, 3, 6, 6] k = 2 print(solve(nums, k))
Input
[8, 6, 6, 4, 3, 6, 6], 2
Output
6