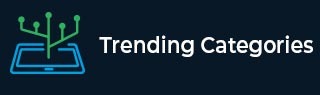
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find length of longest increasing subsequence in Python
Suppose we have a list of numbers. We have to find the length of longest increasing subsequence. So if the input is like [6, 1, 7, 2, 8, 3, 4, 5], then the output will be 5, as the longest increasing subsequence is [2,3,4,5,6].
To solve this, we will follow these steps −
Make an array called tails whose size is same as nums, and fill this with 0.
size := 0
for each element x in nums array −
i := 0, j := size
while i is not same as j, then
mid := i + (j – i)/2
if tails[mid] < x, then i := mid + 1 otherwise j := mid
tails[i] := x
size := max ofi + 1 and size
return size.
Let us see the following implementation to get better understanding −
Example
class Solution(object): def solve(self, nums): tails =[0 for i in range(len(nums))] size = 0 for x in nums: i=0 j=size while i!=j: mid = i + (j-i)//2 if tails[mid]> x: i= mid+1 else: j = mid tails[i] = x size = max(i+1,size) return size ob = Solution() nums = [7, 2, 8, 3, 9, 4, 5, 6] print(ob.solve(nums))
Input
[7, 2, 8, 3, 9, 4, 5, 6]
Output
5
Advertisements