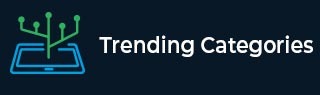
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find length of longest contiguous sublist with same first letter words in Python
Suppose we have a list of lowercase alphabet strings called words. We have to find the length of the longest contiguous sublist where the first letter of each words have the same first letter.
So, if the input is like words = ["she", "sells", "seashells", "on", "the", "sea", "shore"], then the output will be 3, the longest contiguous sublist is ["she", "sells", "seashells"]. The first letter for each words is 's'.
To solve this, we will follow these steps −
cnt := 1
maxcnt := 0
prev_char := blank string
for each word in words, do
if prev_char is empty, then
prev_char := first letter of word
otherwise when prev_char is same as first letter of word, then
cnt := cnt + 1
otherwise,
prev_char := first letter of word
cnt := 1
maxcnt := maximum of maxcnt and cnt
return maxcnt
Example
Let us see the following implementation to get better understanding
def solve(words): cnt = 1 maxcnt = 0 prev_char = "" for word in words: if prev_char == "": prev_char = word[0] elif prev_char == word[0]: cnt += 1 else: prev_char = word[0] cnt = 1 maxcnt = max(maxcnt, cnt) return maxcnt words = ["she", "sells", "seashells", "on", "the", "sea", "shore"] print(solve(words))
Input
["she", "sells", "seashells", "on", "the", "sea", "shore"]
Output
3