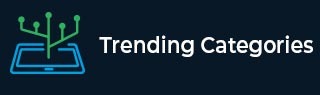
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find length of longest consecutive sequence in Python
Suppose we have an unsorted array of numbers, we have to find the length of the longest sequence of consecutive elements.
So, if the input is like nums = [70, 7, 50, 4, 6, 5], then the output will be 4, as the longest sequence of consecutive elements is [4, 5, 6, 7]. so we return its length: 4.
To solve this, we will follow these steps −
nums := all unique elements of nums
max_cnt := 0
for each num in nums, do
if num - 1 not in nums, then
cnt := 0
while num is present in nums, do
num := num + 1
cnt := cnt + 1
max_cnt := maximum of max_cnt and cnt
return max_cnt
Let us see the following implementation to get better understanding −
Example
class Solution: def solve(self, nums): nums = set(nums) max_cnt = 0 for num in nums: if num - 1 not in nums: cnt = 0 while num in nums: num += 1 cnt += 1 max_cnt = max(max_cnt, cnt) return max_cnt ob = Solution() nums = [70, 7, 50, 4, 6, 5] print(ob.solve(nums))
Input
[70, 7, 50, 4, 6, 5]
Output
4
Advertisements