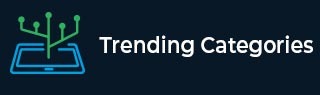
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find largest substring between two equal characters in Python
Suppose we have a string s, we have to find the length of the longest substring between two equal letters or elements, excluding the two characters. If we cannot find such substring, then return -1.
So, if the input is like s = "level", then the output will be 3 as optimal substrings can be either "lev" or "vel".
To solve this, we will follow these steps −
memo := a new map
for i in range 0 to size of s - 1, do
if s[i] is in memo, then
insert i at the end of memo[s[i]]
otherwise,
memo[s[i]] := a list with only one element i
best := 0
for each key in memo, do
best := maximum of best and (last element of memo[key] - first element of memo[key])
return best - 1
Example (Python)
def solve(s): memo = {} for i in range(len(s)): if s[i] in memo: memo[s[i]].append(i) else: memo[s[i]] = [i] best = 0 for key in memo: best = max(best, memo[key][-1] - memo[key][0]) return best - 1 s = "level" print(solve(s))
Input
"level"
Output
3
Advertisements