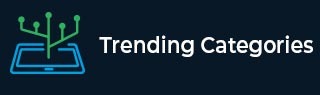
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find largest size to truncate logs to store them completely in database in Python
Suppose we have a list of numbers called logs and another value limit. Each element in logs[i] represents the size of logs generated by the i-th user. And limit represents the total size of logs we can store in our database. We have to find the largest x such that if we truncate every log in logs to be at most size x, and the sum of the left log sizes is at most limit. If no log needs to be truncated, then simply return the largest log size.
So, if the input is like logs = [500, 200, 10000, 500, 4000] limit = 3000, then the output will be 900, because we truncate logs to 900, then we can get [500, 200, 900, 500, 900] now the sum is 3000
To solve this, we will follow these steps −
- lo := 0
- hi := 1 + maximum of logs
- while lo + 1 < hi, do
- mi := lo + floor of (hi - lo)/2
- if sum of all elements present in the list with (minimum of mi and log for each log in logs) <= limit, then
- lo := mi
- otherwise,
- hi := mi
- return lo
Example
Let us see the following implementation to get better understanding −
def solve(logs, limit): lo, hi = 0, max(logs) + 1 while lo + 1 < hi: mi = lo + (hi - lo) // 2 if sum(min(mi, log) for log in logs) <= limit: lo = mi else: hi = mi return lo logs = [500, 200, 10000, 500, 4000] limit = 3000 print(solve(logs, limit))
Input
[500, 200, 10000, 500, 4000], 3000
Output
900