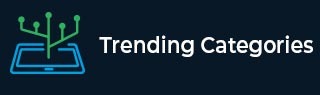
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find largest product of three unique items in Python
Suppose we have a list of numbers called nums, we have to find the largest product of three unique elements.
So, if the input is like nums = [6, 1, 2, 4, -3, -4], then the output will be 72, as we can multiply (- 3) * (-4) * 6 = 72.
To solve this, we will follow these steps −
sort the list nums
n := size of nums
maxScore := -inf
maxScore := maximum of maxScore and (nums[0] * nums[1] * nums[n - 1])
maxScore := maximum of maxScore and (nums[n - 3] * nums[n - 2] * nums[n - 1])
return maxScore
Example
Let us see the following implementation to get better understanding
def solve(nums): nums.sort() n = len(nums) maxScore = -10000 maxScore = max(maxScore, nums[0] * nums[1] * nums[n - 1]) maxScore = max(maxScore, nums[n - 3] * nums[n - 2] * nums[n - 1]) return maxScore nums = [6, 1, 2, 4, -3, -4] print(solve(nums))
Input
[6, 1, 2, 4, -3, -4]
Output
72
Advertisements