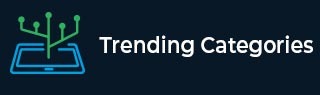
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find indices or local peaks in Python
Suppose we have a list of numbers called nums. We have to find the index of every peak element in the nums, sorted in ascending order. An index i of a peak element when all of these three conditions are satisfied: 1. The next number on its right that's different than nums[i] doesn't present or must be smaller than nums[i] 2. The previous number on its left that's different than nums[i] doesn't present or must be smaller than nums[i] 3. There is at least one number on its left side or right side that is different from nums[i].
So, if the input is like nums = [5, 8, 8, 8, 6, 11, 11], then the output will be [1, 2, 3, 5, 6], because the plateau of 8s are considered as peaks [1,2,3]. And 11s are also, [5, 6].
To solve this, we will follow these steps −
- n := size of nums
- ans := a new list
- i := 0
- while i < n, do
- i0 := i
- while i < n and nums[i] is same as nums[i0], do
- i := i + 1
- if (i0 is 0 or nums[i0] > nums[i0 - 1]) and (i is n or nums[i0] > nums[i]), then
- if i0 is not 0 or i is not n, then
- insert a (list from i0 to i-1) at the end of ans
- if i0 is not 0 or i is not n, then
- return ans
Example
Let us see the following implementation to get better understanding −
def solve(nums): n = len(nums) ans = [] i = 0 while i < n: i0 = i while i < n and nums[i] == nums[i0]: i += 1 if (i0 == 0 or nums[i0] > nums[i0 - 1]) and (i == n or nums[i0] > nums[i]): if i0 != 0 or i != n: ans.extend(range(i0, i)) return ans nums = [5, 8, 8, 8, 6, 11, 11] print(solve(nums))
Input
[5, 8, 8, 8, 6, 11, 11]
Output
[1, 2, 3, 5, 6]