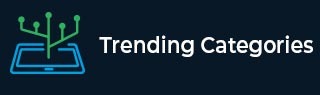
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find concatenation of consecutive binary numbers in Python
Suppose we have a number n, we have to find the decimal value of the binary string by concatenating the binary representations of 1 to n one by one in order, if the answer is too large then return answer modulo 10^9 + 7.
So, if the input is like n = 4, then the output will be 220 because, by concatenating binary representation from 1 to 4 will be "1" + "10" + "11" + "100" = 110111000, this is binary representation of 220.
To solve this, we will follow these steps −
- ans := 1
- m := 10^9+7
- for i in range 2 to n, do
- ans := shift ans (bit length of i) number of times
- ans := (ans+i) mod m
- return ans
Example
Let us see the following implementation to get better understanding −
def solve(n): ans = 1 m = (10**9+7) for i in range(2,n+1): ans = ans<<i.bit_length() ans = (ans+i) % m return ans n = 4 print(solve(n))
Input
4
Output
220
Advertisements