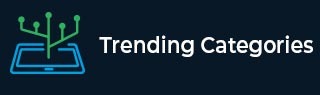
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to find bitwise AND of range of numbers in given range in Python
Suppose we have two values start and end, we have to find the bitwise AND of all numbers in the range [start, end] (both inclusive).
So, if the input is like start = 8 end = 12, then the output will be 8 is 1000 in binary and 12 is 1100 in binary, so 1000 AND 1001 AND 1010 AND 1011 AND 1100 is 1000 which is 8.
To solve this, we will follow these steps −
- n := end - start + 1
- x := 0
- for b in range 31 to 0, decrease by 1, do
- if 2^b < n, then
- come out from loop
- if 2^b AND start AND end is non-zero, then
- x := x + (2^b)
- if 2^b < n, then
- return x
Example
Let us see the following implementation to get better understanding −
def solve(start, end): n = end - start + 1 x = 0 for b in range(31, -1, -1): if (1 << b) < n: break if (1 << b) & start & end: x += 1 << b return x start = 8 end = 12 print(solve(start, end))
Input
8, 12
Output
8
Advertisements