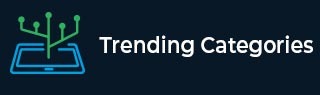
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to expand string represented as n(t) format in Python
Suppose we have a string s, this is encoding a longer string. s is represented as the concatenation of n(t), n(t) represents the concatenation of t, n times, and t is either a regular string or it is another encoded string recursively. We have to find the decoded version of s.
So, if the input is like s = "3(pi)2(3(am))0(f)1(u)", then the output will be "pipipiamamamamamamu"
To solve this, we will follow these steps −
i := 0
Define a function parse() . This will take
ans := a new list
while i < size of s and s[i] is not same as ")", do
if s[i] is a digit, then
d := 0
while s[i] is digit, do
d := 10 * d + integer part of s[i]
i := i + 1
i := i + 1
segment := parse()
i := i + 1
insert segment d times into ans
otherwise,
insert s[i] at the end of ans
i := i + 1
return string after joining items of ans
From the main method return parse()
Example
Let us see the following implementation to get better understanding −
class Solution: def solve(self, s): i = 0 def parse(): nonlocal i ans = [] while i < len(s) and s[i] != ")": if s[i].isdigit(): d = 0 while s[i].isdigit(): d = 10 * d + int(s[i]) i += 1 i += 1 segment = parse() i += 1 ans.extend(segment for _ in range(d)) else: ans.append(s[i]) i += 1 return "".join(ans) return parse() ob = Solution() s = "3(pi)2(3(am))0(f)1(u)" print(ob.solve(s))
Input
"3(pi)2(3(am))0(f)1(u)"
Output
pipipiamamamamamamu