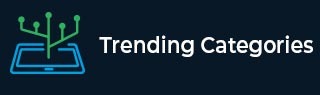
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to evaluate ternary expression in C++
Suppose we have an expression that holds the ternary expression, we have to evaluate the result of the expression. It supports some values like T and F for True and False and “?” and “:” characters. There are some properties:
- The length of the given string must be less than or equal to 10000.
- The conditional expressions group right-to-left.
- The condition will always be either T or F. So the condition will never be a digit.
- The result of the expression will always evaluate to T or F.
So for example, if the input is like “T ? T ? F : T : T”, so the output will be F.
To solve this, we will follow these steps:
- ret := an empty string, n := size of s,
- create a stack st
- for i in range n – 1 down to 0
- x := s[i]
- if st is not empty and top of stack is ‘?’, then
- delete from st
- first := top of st, then delete two elements from stack
- second := top of stack, and delete from st
- if x is T, then insert first into st, otherwise insert second into st
- otherwise insert x into st
- while st is not empty, then
- ret := ret + top of st and delete from st
- reverse ret and return ret
Let us see the following implementation to get better understanding:
Example
#include using namespace std; class Solution { public: string parseTernary(string s) { string ret = ""; int n = s.size(); stack st; for(int i = n - 1; i >= 0; i--){ char x = s[i]; if(!st.empty() && st.top() == '?'){ st.pop(); char first = st.top(); st.pop(); st.pop(); char second = st.top(); st.pop(); if(x == 'T'){ st.push(first); } else st.push(second); } else{ st.push(x); } } while(!st.empty()){ ret += st.top(); st.pop(); } reverse(ret.begin(), ret.end()); return ret; } }; main(){ Solution ob; cout << (ob.parseTernary("T?T?F:T:T")); }
Input
" T?T?F:T:T"
Output
F
Advertisements