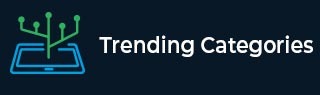
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to count number of operations needed to make string as concatenation of same string twice in Python
Suppose we have a lowercase string s. Now consider an operation, where we can delete, insert or update any character in s. We have to count minimum number of operations required to make s = (t concatenate t) for any string t.
So, if the input is like s = "pqrxqsr", then the output will be 2, because, we can update the "x" with "p" and delete "s", then s is "pqrpqr", this is s = t concatenate t, for t = "pqr".
To solve this, we will follow these steps −
- Define a function edit_distance(). This will take s1, s2
- m := size of s1
- n := size of s2
- cur := a new list from range 0 to n
- for i in range 0 to m - 1, do
- prev := cur
- cur := a list with (i + 1) and then n number of 0s
- for j in range 0 to n - 1, do
- cur[j + 1] := prev[j] if s1[i] and s2[j] are same otherwise (minimum of cur[j], prev[j], prev[j + 1]) + 1
- return cur[n]
- From the main method, do the following −
- res := size of s
- for i in range 0 to size of s - 1, do
- res := minimum of edit_distance(substring of s from index 0 to i - 1, substring of s from index i to end) and res
- return res
Example
Let us see the following implementation to get better understanding −
def solve(s): def edit_distance(s1, s2): m, n = len(s1), len(s2) cur = list(range(n + 1)) for i in range(m): prev, cur = cur, [i + 1] + [0] * n for j in range(n): cur[j + 1] = (prev[j] if s1[i] == s2[j] else min(cur[j], prev[j], prev[j + 1]) + 1) return cur[n] res = len(s) for i in range(len(s)): res = min(edit_distance(s[:i], s[i:]), res) return res s = "pqrxqsr" print(solve(s))
Input
"pqrxqsr"
Output
None
Advertisements