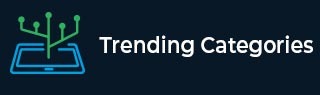
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to count nice pairs in an array in Python
Suppose we have an array called nums with non-negative values. We have to find number nice pairs of indices present in the array, if the answer is too large, then return answer mod 10^9+7. Here a pair of indices (i, j) is said to be nice when they satisfy all of these conditions: 1. 0 <= i < j < size of nums 2. nums[i] + rev(nums[j]) is same as nums[j] + rev(nums[i])
Note − Here rev() reverses only the positive part of an integer, so if rev(564) is there, it means 465, but if rev(540) is there, it returns 45.
So, if the input is like nums = [97,2,42,11], then the output will be 2 because there are two pairs (0,2) and (1,3), for the first one [97 + rev(42) = 97+24 = 121, and 42 + rev(97) = 42 + 79 = 121] and for the second one [2 + rev(11) = 2 + 11 = 13 and 11 + rev(2) = 13].
To solve this, we will follow these steps −
m :=(10^9) +7
dic := an empty map, whose default value is 0
for each num in nums, do
rev := reverse of num
increase dic[num - rev] by 1
res:= 0
for each val in list of all values of dic, do
res := res + quotient of (val*(val-1))/2
return res mod m
Example
Let us see the following implementation to get better understanding −
from collections import defaultdict def solve(nums): m = (10**9)+7 dic = defaultdict(int) for num in nums: rev=int(str(num)[::-1]) dic[num-rev]+=1 res=0 for val in dic.values(): res += (val*(val-1)) // 2 return res % m nums = [97,2,42,11] print(solve(nums))
Input
[97,2,42,11]
Output
2