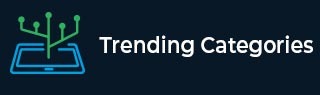
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to convert KiloBytes to Bytes and Bits in C++
Given with the input as KiloBytes and the task is to convert the given input into number of bytes and bits.
Bit − In computers, bit is the smallest unit represented with two integer value 0 and 1 and all the information in computer is processed as a sequence of these two digits.
N-bits = 2 ^ N patterns, where N can be any integer value starting from 1.
Byte − In computers, byte is represented with 8 bits. Byte is capable of holding one character to numbers ranging between 0 and 255.
1 byte = 8 bits
Which means 2 ^ 8 patterns which is equals to 256
There are multiple forms of bytes −
1 Kilobyte(KB) = 1024 bytes
1 Megabyte(MB) = 1048576 bytes
1 Gigabyte = 1073741824 bytes
Example
Input 1-: kilobytes = 10 Output -: 10 Kilobytes = 10240 Bytes and 81920 Bits Input 2-: kilobytes = 1 Output -: 1 Kilobytes = 1024 Bytes and 8192 Bits
Approach used below is as follows −
- Input data in kilobytes
Apply the formula to convert the kilobytes into bytes
Bytes = kilobytes * 1024
Apply the formula to convert the kilobytes into bits
Bits = kilobytes * 8192
Algorithm
Start Step 1-> Declare function to convert into bits long Bits(int kilobytes) set long Bits = 0 set Bits = kilobytes * 8192 return Bits step 2-> Declare function to convert into bytes long Bytes(int kilobytes) set long Bytes = 0 set Bytes = kilobytes * 1024 return Bytes step 3-> In main() declare int kilobytes = 10 call Bits(kilobytes) call Bytes(kilobytes) Stop
Example
#include <bits/stdc++.h> using namespace std; //convert into bits long Bits(int kilobytes) { long Bits = 0; Bits = kilobytes * 8192; return Bits; } //convert into bytes long Bytes(int kilobytes) { long Bytes = 0; Bytes = kilobytes * 1024; return Bytes; } int main() { int kilobytes = 10; cout << kilobytes << " Kilobytes = " << Bytes(kilobytes) << " Bytes and " << Bits(kilobytes) << " Bits"; return 0; }
Output
IF WE RUN THE ABOVE CODE IT WILL GENERATE FOLLOWING OUTPUT
10 Kilobytes = 10240 Bytes and 81920 Bits