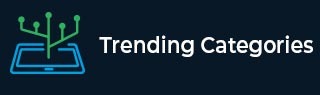
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to check whether we can make group of two partitions with equal sum or not in Python?
Suppose we have a list of numbers called nums, we have to check whether we can partition nums into two groups where the sum of the elements in both groups are same.
So, if the input is like nums = [2, 3, 6, 5], then the output will be True, as we can make groups like: [2, 6] and [3, 5].
To solve this, we will follow these steps
total := sum of all elements in nums
if total is odd, then
return False
half := integer part of total / 2
dp := a list of size half + 1 and fill with false
dp[0] := true
for each num in nums, do
for i in range half to 0, decrease by 1, do
if i >= num, then
dp[i] := dp[i] OR dp[i - num]
return dp[half]
Example
class Solution: def solve(self, nums): total = sum(nums) if total & 1: return False half = total // 2 dp = [True] + [False] * half for num in nums: for i in range(half, 0, -1): if i >= num: dp[i] |= dp[i - num] return dp[half] ob = Solution() nums = [2, 3, 6, 5] print(ob.solve(nums))
Input
[2, 3, 6, 5]
Output
True
Advertisements