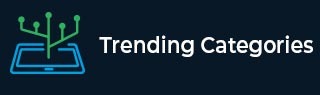
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to check whether we can form 24 by placing operators in python
Suppose we have a list of four numbers, each numbers are in range 1 to 9, in a fixed order. Now if we place the operators +, -, *, and / (/ denotes integer division) between the numbers, and group them with brackets, we have to check whether it is possible to get the value 24 or not.
So, if the input is like nums = [5, 3, 6, 8, 7], then the output will be True, as (5 * 3) - 6 + (8 + 7) = 24.
To solve this, we will follow these steps −
- Define a function recur() . This will take arr
- answer := a new list
- for i in range 0 to size of arr - 1, do
- pre := recur(arr[from index 0 to i])
- suf := recur(arr[from index i + 1 to end])
- for each k in pre, do
- for each j in suf, do
- insert (k + j) at the end of answer
- insert (k - j) at the end of answer
- insert (k * j) at the end of answer
- if j is not 0, then
- insert (quotient of k / j) at the end of answer
- for each j in suf, do
- if size of answer is 0 and size of arr is 1, then
- insert arr[0] at the end of answer
- return answer
- From the main method check whether 24 is in recur(nums) or not, if yes return True, otherwise false
Let us see the following implementation to get better understanding −
Example
class Solution: def solve(self, nums): def recur(arr): answer = [] for i in range(len(arr) - 1): pre, suf = recur(arr[: i + 1]), recur(arr[i + 1 :]) for k in pre: for j in suf: answer.append(k + j) answer.append(k - j) answer.append(k * j) if j != 0: answer.append(k // j) if len(answer) == 0 and len(arr) == 1: answer.append(arr[0]) return answer return 24 in recur(nums) ob = Solution() nums = [5, 3, 6, 8, 7] print(ob.solve(nums))
Input
[5, 3, 6, 8, 7]
Output
True
Advertisements