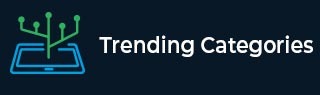
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to check whether first player can take more candies than other or not in Python
Suppose we have a list of numbers called candies and two persons are racing to collect the most number of candies. Here the race is turn based, person 1 is starting first, and in each turn he can pick up candies from the front or from the back. We have to check whether person 1 can collect more candies than other or not.
So, if the input is like candies = [1, 4, 3, 8], then the output will be True, as person 1 can take 8 candies in the first round and regardless of whether second person picks 1 or 3, person 1 can win by taking any remaining candy.
To solve this, we will follow these steps −
N := size of candies
Define a function difference() . This will take left, right
if left is same as right, then
return candies[left]
return maximum of (candies[left] − difference(left + 1, right)) and (candies[right] − difference(left, right − 1))
from the main method do the following −
return true when difference(0, N − 1) > 0, otherwise false
Let us see the following implementation to get better understanding −
Example
class Solution: def solve(self, candies): N = len(candies) def difference(left, right): nonlocal candies if left == right: return candies[left] return max(candies[left] − difference(left + 1, right), candies[right] − difference(left, right − 1)) return difference(0, N − 1) > 0 ob = Solution() candies = [1, 4, 3, 8] print(ob.solve(candies))
Input
[1, 4, 3, 8]
Output
True