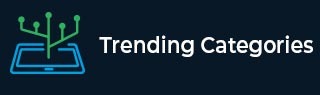
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to check we can rearrange array to make difference between each pair of elements same in Python
Suppose we have a list called nums, we have to check whether we can rearrange the order of nums in such a way that the difference between every pair of consecutive two numbers is same.
So, if the input is like nums = [8, 2, 6, 4], then the output will be True, because if we rearrange nums like [2, 4, 6, 8], then the difference between every two pair of consecutive numbers is 2.
To solve this, we will follow these steps −
N := size of nums
if N <= 2, then
return True
sort the list nums
targetDiff := nums[1] - nums[0]
for i in range 2 to N - 1, do
if nums[i] - nums[i - 1] is not same as targetDiff, then
return False
return True
Example
Let us see the following implementation to get better understanding
def solve(nums): N = len(nums) if N <= 2: return True nums.sort() targetDiff = nums[1] - nums[0] for i in range(2, N): if nums[i] - nums[i - 1] != targetDiff: return False return True nums = [8, 2, 6, 4] print(solve(nums))
Input
[8, 2, 6, 4]
Output
True
Advertisements