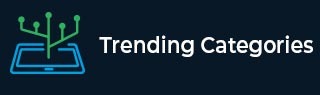
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Program to check if water tank overflows when n solid balls are dipped in the water tank in C++
Given with the radius and height of cylindrical water tank, ‘n’ number of spherical solid balls with the radius and the volume of water in the tank and the task is to check whether the tank will overflow or not when balls are dipped in the tank.
Formula to calculate the volume
Cylinder
3.14 * r * r * h
Where, r is radius of tank and h is the height of the tank
Sphere
(4/3) * 3.14 * R * R * R
Where, R is radius of sphere ball
Input
tank_height = 5 tank_radius = 2 water_volume = 10 capacity = 10 ball_radius = 2
Output
It will overflow
Approach used below is as follows
Input the given dimensions like radius of tank, height of tank, number of balls to be dipped and radius of shere
Calculate the capacity (volume) of a tank by applying the formula
Calculate the volume of a sphere by applying its formula
Calculate the volume of a water as the volume will increase whenever the ball is dipped into a water tank.
Calculate the total volume by adding the volume of water and volume of sphere
Check the condition to state whether the tank will overflow or not
If the total volume is greater than capacity than tank will overflow
If the total volume is lesser than capacity than tank will not overflow
Algorithm
Step 1→ declare function to check whether tank will overflow or not void overflow(int H, int r, int h, int N, int R) declare float tank_cap = 3.14 * r * r * H declare float water_vol = 3.14 * r * r * h declare float balls_vol = N * (4 / 3) * 3.14 * R * R * R declare float vol = water_vol + balls_vol IF (vol > tank_cap) Print it will overflow End Else Print No it will not overflow End Step 2→ In main() Declare int tank_height = 5, tank_radius = 2, water_volume = 10, capacity = 10, ball_radius = 2 call overflow(tank_height, tank_radius, water_volume, capacity, ball_radius)
Example
#include <bits/stdc++.h> using namespace std; //check whether tank will overflow or not void overflow(int H, int r, int h, int N, int R){ float tank_cap = 3.14 * r * r * H; float water_vol = 3.14 * r * r * h; float balls_vol = N * (4 / 3) * 3.14 * R * R * R; float vol = water_vol + balls_vol; if (vol > tank_cap){ cout<<"it will overflow"; } else{ cout<<"No it will not overflow"; } } int main(){ int tank_height = 5, tank_radius = 2, water_volume = 10, capacity = 10, ball_radius = 2; overflow(tank_height, tank_radius, water_volume, capacity, ball_radius); return 0; }
Output
If run the above code it will generate the following output −
it will overflow