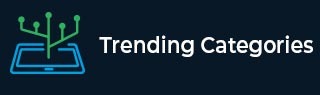
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Probability of getting at least K heads in N tosses of Coins in C++
Probability is the chances of getting the desired output from the set of data available. The range of probability lie between 0 and 1 where an integer 0 shows the chances of impossibility and 1 indicates certainty.
What is probability?
Probability in mathematics gives us the tools that tell the uncertainty of the events and reasons. In other words we can say probability deals with calculating the likelihood of a given event’s occurrence, which can be expressed as a number between 1 and 0. For example: the probability of getting a head’s when an unbiased coin is tossed, or getting a 3 when a dice is rolled.
Now, coming back to the question we have to find the probability of getting at least k heads in N tosses of coins.
Like we have 3 coins and k as 2 so there are23= 8 ways to toss the coins that is −
HHH, HTH, HHT, HTT, THH, THT, TTT, TTH.
And the sets which contains at least 2 heads are −
HHH, HTH, HHT, THH.
So the probability will be 4/8 or 0.5.
Example
Input: k = 1, n = 3 Output: 0.875 Input: k = 3, n = 6 Output: 0.65625
Approach we will be following to solve the above problem −
- We will take n and k as an input.
- Store the value of factorial in an array and call it whenever it is required.
- Perform the calculation.
- Return the result.
Algorithm
Step 1-> declare function to calculate the probability of getting at least k heads in n tosses double probability(int k, int n) Declare and set double check = 0 Loop For i = k and i <= n and ++i Set check += temp[n] / (temp[i] * temp[n - i]) End Call check = check / (1LL << n) return check Step 2-> declare function to precompute the value void precompute() Set temp[0] = temp[1] = 1 Loop For i = 2 and i < 20 and ++i Set temp[i] = temp[i - 1] * i Step 3-> In main Call precompute() Call probability(1, 3) Stop
Example
#include<bits/stdc++.h> using namespace std; #define size 21 double temp[size]; // calculate probability of getting at least k heads in n tosses. double probability(int k, int n) { double check = 0; for (int i = k; i <= n; ++i) check += temp[n] / (temp[i] * temp[n - i]); check = check / (1LL << n); return check; } void precompute() { temp[0] = temp[1] = 1; for (int i = 2; i < 20; ++i) temp[i] = temp[i - 1] * i; } int main() { precompute(); // Probability of getting 1 head out of 3 coins cout<<"probability is : "<<probability(1, 3) << "\n"; // Probability of getting 3 head out of 6 coins cout<<"probability is : "<<probability(3, 6) <<"\n"; return 0; }
Output
probability is : 0.875 probability is : 0.65625