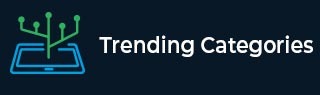
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Probability of a random pair being the maximum weighted pair in C++
Given with two different arrays and the task is to find the probability of the random pair chosen to be the maximum weighted pair.
A Pair will contain one element from let’s say array1 and another element form another array let’s say array2. So the program must find the probability of the pair that will contain first element to be the maximum element of array 1 and second element to be the maximum element of array 2 hence forming the maximum weighted pair.
Input
arr1[] = { 2, 23 } arr2[] = { 10, 3, 8 }
Output
probability of maximum pair : 0.166667
Explanation
set of pairs from both the arrays are -: {(2, 10), (2, 3), (2, 8), (23, 10), (23, 3), (23, 8)} Maximum weighted pair from the given set is: (23, 8) Probability is : 1 / 6 = 0.166667
Input −
arr1[] = { 4, 5, 6 } arr2[] = { 6, 2, 6 }
Output −
probability of maximum pair : 0.222222
Explanation
set of pairs from both the arrays are -: {(4, 6), (4, 2), (4, 6), (5, 6), (5, 2), (5, 6), (6, 6), (6, 2), (6, 6)} Maximum weighted pair from the given set is (6, 6) which is occurring twice. Probability is : 2 / 9 = 0.2222
Approach
Input the array elements
Find the maximum elements from both the arrays and calculate the total number of maximum weighted pairs so formed
Calculate the probability by dividing the total number of maximum weighted pairs with the total number of pairs in the set
Print the calculated probability
Algorithm
Start Step1→ declare function to calculate maximum weighted pair double max_pair(int arr1[], int arr2[], int size_1, int size_2) declare int max_pair1 = INT_MIN, count_1 = 0 Loop For int i = 0 and i < size_1 and i++ IF arr1[i] > max_pair1 Set max_pair1 = arr1[i] Set count_1 = 1 End Else IF arr1[i] = max_pair1 Set count_1++ End End Declare int max_pair2 = INT_MIN, count_2 = 0 Loop For int i = 0 and i < size_2 and i++ IF arr2[i] > max_pair2 Set max_pair2 = arr2[i] Set count_2 = 1 End Else IFarr2[i] = max_pair2 Set count_2++ End End return (double)(count_1 * count_2) / (size_1 * size_2) Step 2→ In main() Declare int arr1[] = { 2, 23 } Declare int arr2[] = { 10, 3, 8 } Calculate int size_1 = sizeof(arr1) / sizeof(arr1[0]) Calculate int size_2 = sizeof(arr2) / sizeof(arr2[0]) Call max_pair(arr1, arr2, size_1, size_2) Stop
Example
#include <bits/stdc++.h> using namespace std; // Function to return probability double max_pair(int arr1[], int arr2[], int size_1, int size_2){ //pair from array 1 int max_pair1 = INT_MIN, count_1 = 0; for (int i = 0; i < size_1; i++){ if (arr1[i] > max_pair1){ max_pair1 = arr1[i]; count_1 = 1; } else if (arr1[i] == max_pair1){ count_1++; } } //pair from array 2 int max_pair2 = INT_MIN, count_2 = 0; for (int i = 0; i < size_2; i++){ if (arr2[i] > max_pair2){ max_pair2 = arr2[i]; count_2 = 1; } else if (arr2[i] == max_pair2){ count_2++; } } return (double)(count_1 * count_2) / (size_1 * size_2); } int main(){ int arr1[] = { 2, 23 }; int arr2[] = { 10, 3, 8 }; int size_1 = sizeof(arr1) / sizeof(arr1[0]); int size_2 = sizeof(arr2) / sizeof(arr2[0]); cout <<"probability of maximum pair in both the arrays are "<<max_pair(arr1, arr2, size_1, size_2); return 0; }
Output
If run the above code it will generate the following output −
probability of maximum pair in both the arrays are 0.166667