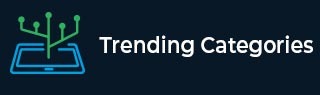
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Print Concatenation of Zig-Zag String in n Rows in C++
In this problem, we are given a string that is a sequence of characters. And we are given the length of the zig-zag pattern and we have to print the concatenation string of this zig-zag string in n rows.
Let’s see a few examples to understand the concept better,
EXAMPLE
Input : string = ‘STUVWXYZ’ n = 2. Output : SUWYTVXZ
Explanation − the zig-zag pattern for the string for a 2-row pattern is −
S U W Y T V X Z
The concatenation of this zig-zag pattern is - SUWYTVXZ.
Example
Input : string = ABCDEFGH n = 3 Output: ADGBEHCF
Explanation − the zigzag pattern for the string with three rows is −
A E B D F H C G
The concatenation of the zigzag pattern is - AEBDFHCG
Now as we know the problem, let's design a solution for it. here we will propagate down and next element of the string till the death becomes n. and then proper get up till the death becomes zero and then again go down. and then print each row for the solution.
Based on this concept let's derive an algorithm that can solve the problem,
Algorithm
Step 1 : Take an array of string of size n, string arr[n], row for current row no. i.e. string in the array and the direction as 1(indicating downward traversal). Step 2 : Traverse the string and follow step 3 - 7. For every character of the string. Step 3 : Append the character to the current string in the array based on the value of row. Step 4 : If row = n-1, direction = 1. Step 5 : if row = 0, direction = -1. Step 6 : if direction = 1, row++ . Step 7 : else row--. Step 8 : print all string on the array from 0 to n-1 in sequence.
Example
Now based on this algorithm create a program to implement our solution −
#include<bits/stdc++.h> using namespace std; void ZigZagConcatenationString(string str, int n){ if (n == 1){ cout << str; return; } int len = str.length(); string arr[n]; int row = 0; int direction = 1; bool down; for (int i = 0; i < len; ++i){ arr[row].push_back(str[i]); if (row == n-1) direction = -1; else if (row == 0) direction = 1; (direction == 1)? (row++): (row--); } for (int i = 0; i < n; ++i) cout << arr[i]; } int main(){ string str = "ABCDEFGH"; int n = 3; ZigZagConcatenationString(str, n); return 0; }
Output
AEBDFHCG