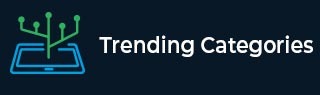
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Print all subarrays with 0 sum in C++
In this problem, we are given an array of integer values and we have to print all those subarrays from this array that have the sum equal to 0.
Let’s take an example to understand the topic better,
Input: array = [-5, 0, 2, 3, -3, 4, -1] Output: Subarray with sum 0 is from 1 to 4. Subarray with sum 0 is from 5 to 7 Subarray with sum 0 is from 0 to 7
To solve this problem, we will check all subarrays possible. And check if the sum of these subarrays is equal to 0 and print them. This solution is easy to understand but the solution is complex, and its time complexity is of the order O(n^2).
A better solution to this problem is using Hashing. For solving this we will find the sum if it equals 0 add it to the Hash table.
Algorithm
Step 1: Create a sum variable. Step 2: If sum =0, subarray starts from index 0 to end index of the array. Step 3: If the current sum is in the hash table. Step 4: If the sum exists, then subarray from i+1 to n must be zero. Step 5: Else insert into the hash table.
Example
#include <bits/stdc++.h> using namespace std; vector< pair<int, int> > findSubArrayWithSumZero(int arr[], int n){ unordered_map<int, vector<int> >map; vector <pair<int, int>> out; int sum = 0; for (int i = 0; i < n; i++){ sum += arr[i]; if (sum == 0) out.push_back(make_pair(0, i)); if (map.find(sum) != map.end()){ vector<int> vc = map[sum]; for (auto it = vc.begin(); it != vc.end(); it++) out.push_back(make_pair(*it + 1, i)); } map[sum].push_back(i); } return out; } int main(){ int arr[] = {-5, 0, 2, 3, -3, 4, -1}; int n = sizeof(arr)/sizeof(arr[0]); vector<pair<int, int> > out = findSubArrayWithSumZero(arr, n); if (out.size() == 0) cout << "No subarray exists"; else for (auto it = out.begin(); it != out.end(); it++) cout<<"Subarray with sum 0 is from "<<it->first <<" to "<<it->second<<endl; return 0; }
Output
Subarray with sum 0 is from 1 to 1 Subarray with sum 0 is from 0 to 3 Subarray with sum 0 is from 3 to 4 Subarray with sum 0 is from 0 to 6 Subarray with sum 0 is from 4 to 6
Advertisements