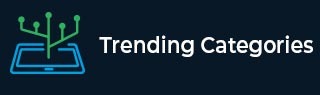
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Print all pairs with given sum in C++
In this problem, we are given an array of integers and an integer sum and we have to print all pairs of integers whose sum is equal to the sum value.
Let’s take an example to understand the problem :
Input − array = {1, 6, -2, 3} sum = 4
Output − (1, 3) , (6, -2)
Here, we need pairs with the given sum value.
A simple solution to the problem will be checking pairs of elements that generate the sum. This can be done by traversing array and find the number in array that sums up to sum value.
Example
This program will illustrate the solution −
#include <iostream> using namespace std; int printPairsWithSum(int arr[], int n, int sum){ int count = 0; for (int i = 0; i < n; i++) for (int j = i + 1; j < n; j++) if (arr[i] + arr[j] == sum) cout<<"[ "<<arr[i]<<", "<<arr[j]<<" ]\n"; } int main(){ int arr[] = {1, 6, -2, 3}; int n = 4; int sum = 4; cout<<"Pairs with Sum "<<sum<<" are :\n"; printPairsWithSum(arr, n, sum); return 0; }
Output
Pairs with Sum 4 are : [ 1, 3 ] [ 6, -2 ]
This method is easy to understand but not quite efficient. Another way will be using hashing.
We will initialise a hash table and traverse the array and find pairs in it. On match, we will print the array :
Example
The following program will make you understand the algorithm better −
#include <bits/stdc++.h> using namespace std; void printPairsWithSum(int arr[], int n, int sum){ unordered_map<int, int> pair; for (int i = 0; i < n; i++) { int rem = sum - arr[i]; if (pair.find(rem) != pair.end()) { int count = pair[rem]; for (int j = 0; j < count; j++) cout<<"["<<rem<<", "<<arr[i]<<" ]\n"; } pair[arr[i]]++; } } int main(){ int arr[] = {1, 6, -2, 3}; int n = 4; int sum = 4; cout<<"The pair with sum is \n"; printPairsWithSum(arr, n, sum); return 0; }
Output
Pairs with Sum 4 are : [ 1, 3 ] [ 6, -2 ]