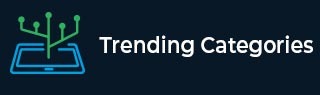
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Print all distinct permutations of a given string with duplicates in C++
In this problem, we are given a string that may contain duplicate characters. Our task is to print all distinct permutations of the strings.
Let’s take an example to understand the problem −
Input: string = “XYZ” Output: XYZ XZY YXZ YZX ZYX ZXY
To solve this problem, we have to fix one element of the string. And then iterate all the elements of the strings.
Example
Program to implement our solution,
#include <string.h> #include <iostream> using namespace std; int compare(const void* a, const void* b) { return (*(char*)a - *(char*)b); } void swapChar(char* a, char* b) { char t = *a; *a = *b; *b = t; } int findCeil(char str[], char first, int l, int h) { int ceilIndex = l; for (int i = l + 1; i <= h; i++) if (str[i] > first && str[i] < str[ceilIndex]) ceilIndex = i; return ceilIndex; } void printPermutations(char str[]) { int size = strlen(str); qsort(str, size, sizeof(str[0]), compare); bool isFinished = false; while (!isFinished) { static int x = 1; cout<<str<<"\t"; int i; for (i = size - 2; i >= 0; --i) if (str[i] < str[i + 1]) break; if (i == -1) isFinished = true; else { int ceilIndex = findCeil(str, str[i], i + 1, size - 1); swapChar(&str[i], &str[ceilIndex]); qsort(str + i + 1, size - i - 1, sizeof(str[0]), compare); } } } int main() { char str[] = "SNGY"; cout<<"All permutations of the string"<<str<<" are :\n"; printPermutations(str); return 0; }
Output
All permutations of the stringSNGY are − GNSY GNYS GSNY GSYN GYNS GYSN NGSY NGYS NSGY NSYG NYGS NYSG SGNY SGYN SNGY SNYG SYGN SYNG YGNS YGSN YNGS YNSG YSGN YSNG
Advertisements