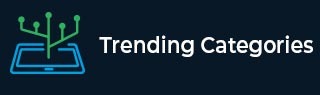
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Print a pattern without using any loop in C++
In this problem, we are given a number n. Our task is to print patterns with decreasing to 0 or negative then increasing back to the number.
Let’s take an example to understand the problem,
Input: n = 12 Output: 12 7 2 -3 2 7 12
To solve this problem, we will use recursion and call the function after each update. The track of update is kept using the flag variable which tells the function to increase or decrease the number by 5.
Example
The below code gives the implementation of our solution,
#include <iostream> using namespace std; void printNextValue(int m){ if (m > 0){ cout<<m<<'\t'; printNextValue(m - 5); } cout<<m<<'\t'; } int main(){ int n = 13; cout<<"The pattern is:\n"; printNextValue(n); return 0; }
Output
The pattern is − 13 8 3 -2 3 8 13
Advertisements