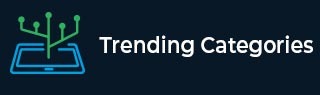
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Print a number as string of 'A' and 'B' in lexicographic order in C++
In this problem, we are given a number N. Our task is to create a program to Print a number as string of ’A’ and ‘B’ in lexicographic order.
Representation of all numbers as string of ‘A’ and ‘B’ is
1 = A
2 = B
3 = AA
4 = AB
5 = BA
6 = BB
7 = AAA
8 = AAB
Let’s take an example to understand the problem,
Input: N = 12
Output: BAB
Solution Approach
The string of ‘A’ and ‘B’ is similar to a binary number. To find the string, we will first find the length of string, using the fact that there are 2 numbers of length 1 (till size 2), 4 numbers of length 2 (till size 6), 8 numbers of length 3 (till size 14). After finding the length we need to find the characters of the string. By iteratively updating the length remaining as we add numbers to the string. The character is decided based on comparing N with (2^(length remaining)), if N is smaller than the current character is ‘B’ otherwise its ‘A’. After each iteration we will decrease the length by 1 and if the character is ‘B’ will update N, and decrease it by num.
Program to illustrate the working of our solution,
Example
#include <iostream> #include<math.h> using namespace std; int findStringLength(int M) { int stringLen = 1; while((pow(2, stringLen + 1) - 2) < M) { stringLen++; } return stringLen; } void printNumString(int N) { int stringLen, num, stringNumber; stringLen = findStringLength(N); stringNumber = N - (pow(2, stringLen) - 2); while (stringLen) { num = pow(2, stringLen - 1); if (num < stringNumber) { cout<<"B"; stringNumber -= num; } else { cout<<"A"; } stringLen--; } } int main() { int N = 47; cout<<"The number as sting of 'A' and 'B' in lexicographic order is "; printNumString(N); return 0; }
Output
The number as sting of 'A' and 'B' in lexicographic order is BAAAA