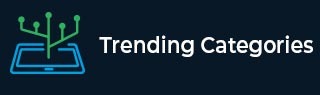
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Previous smaller integer having one less number of set bits in C++
In this problem, we are given an integer n. Our task is to print the largest number less than n which can be formed by changing one set bit of the binary representation of the number.
Let’s take an example to understand the problem
Input: n = 3 Output: 2 Explanation: (3)10 = (011)2 Flipping one set bit gives 001 and 010. 010 is greater i.e. 2.
To solve this problem, we will have to flip the rightmost set bit and make it zero which will create the number the greatest possible number less than n that is found by flipping one bit of the number.
Program to show the implementation of our solution,
Example
#include<iostream> #include<math.h> using namespace std; int returnRightSetBit(int n) { return log2(n & -n) + 1; } void previousSmallerInteger(int n) { int rightBit = returnRightSetBit(n); cout<<(n&~(1<<(rightBit - 1))); } int main() { int n = 3452; cout<<"The number is "<<n<<"\nThe greatest integer smaller than the number is : "; previousSmallerInteger(n); return 0; }
Output
The number is 3452 The greatest integer smaller than the number is : 3448
Advertisements