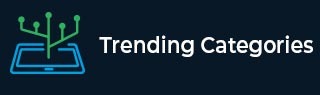
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Powerful Integers in C++
Let us suppose we have three integers 'a' and 'b' and 'limit'. The task is to print the numbers in the range [a, limit]. List of these numbers is said to be powerful integers and represented as,
a^i + b^j such that i >= 0 and j >= 0
For Example
Input-1:
a = 2
b = 5
limit = 10
Output:
[2, 3, 4, 5, 6, 7, 9]
Explanation: for each i and j,
2^0 + 5^0 = 2 , 2^0 + 5^1= 6
2^1 + 5^0 = 3 , 2^1 + 5^1= 7
2^2 + 5^0 =5 , 2^3 + 5^0= 9
Approach to Solve this Problem
The brute force approach to solve this particular problem is that we will take two nested loops and iterate till the limit. Then we will find the sum of two numbers for each exponent in the upper bound and insert the resultant number in the list.
- Take three numbers 'a', 'b' and 'limit'.
- A function powerfulnumbers(int a, int b, int limit) takes the numbers 'a' , 'b' and the 'limit' as the input. The function returns the list of all the powerful numbers such that a^i + b^j where i >= 0 and j >= 0.
- Take two nested loops ranging till the limit and every time, find the powerful numbers by multiplying with its indexes.
- If the number is lying between the range [a, limit], then store the number in a set (to avoid duplicates of the numbers).
- Iterate over the set and print the output.
Example
#include <bits/stdc++.h> using namespace std; void powerfulNum(int a, int b, int limit) { set < int > s; for (int i = 1; i < limit; i *= a) { for (int j = 1; j < limit; j *= b) { if (i + j <= limit) { s.insert(i + j); } else break; if (b == 1) break; } if (a == 1) break; } for (auto it: s) { cout << it << " "; } } int main() { int a = 2; int b = 5; int limit = 10; powerfulNum(a, b, limit); return 0; }
Running the above code will generate the output as,
Output
2 3 5 6 7 9
Here, all the powerful numbers in the range 2 to 10 are [2, 3, 4, 6, 7, 9].