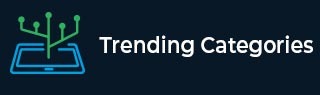
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Postfix to Infix in C++
In this problem, we are given expression in postfix form and our task is to print the infix form of the expression.
Infix expression is an expression in which the operator is in the middle of operands, like operand operator operand.
Postfix expression is an expression in which the operator is after operands, like operand operator.
Postfix expressions are easily computed by the system but are not human readable. So this conversion is required. Generally reading and editing by the end-user is done on infix notations as they are parenthesis separated hence easily understandable for humans.
Let’s take an example to understand the problem
Input − xyz/*
Output − (x * (y/z))
To solve this problem, we will use the stack data structure. And traverse the postfix expression one by one and then check for the following case −
Case 1 − if the operand is found, push it in the stack.
Case 2 − if an operator is found, pop to operands, create an infix expression of the three and push the expression as an operand.
In the end when the stack has only one element left and the traversing is done, pop the top of stack, it is the infix conversion.
Example
Program to show the implementation of our solution.
#include <bits/stdc++.h> using namespace std; bool isOperand(char x) { return (x >= 'a' && x <= 'z') || (x >= 'A' && x <= 'Z'); } string infixConversion(string postfix) { stack<string> infix; for (int i=0; postfix[i]!='\0'; i++) { if (isOperand(postfix[i])) { string op(1, postfix[i]); infix.push(op); } else { string op1 = infix.top(); infix.pop(); string op2 = infix.top(); infix.pop(); infix.push("{"+op2+postfix[i]+op1 +"}"); } } return infix.top(); } int main() { string postfix = "xyae+/%"; cout<<"The infix conversion of the postfix expression '"<<postfix<<"' is : "; cout<<infixConversion(postfix); return 0; }
Output
The infix conversion of the postfix expression 'xyae+/%' is : {x%{y/{a+e}}}