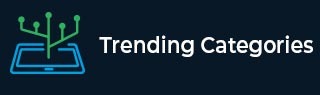
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Possible number of Rectangle and Squares with the given set of elements in C++
In this problem, we are given an array of N integers denoting the length of n sticks. Our task is to print the count of rectangles and squares that can be created from the sticks of the given length.
Let’s take an example to understand the problem
Input − array = {5, 5, 7, 7, 1, 4}
Output − 1
Explanation − a rectangle with sides 5 5 7 7.
To solve this problem, we will have to check if rectangles and squares are possible or not.
Now, for creating a square or rectangle, there should be two sticks of the same length, 2 for rectangle and 4 for square. Now, in our array, we will have to check for pairs of sticks of the same length. For making this search easy, we will sort the array and then find pairs and half of the total pair count will be the number of squares or rectangles that can be created.
Example
Program to show the implementation of our solution,
#include <bits/stdc++.h> using namespace std; int countRecSqr(int sticks[], int n) { sort(sticks, sticks + n); int pairs = 0; for (int i = 0; i < n - 1; i++) { if (sticks[i]==sticks[i + 1]) { pairs++; i++; } } return pairs / 2; } int main() { int sticks[] = { 2, 2, 4, 4, 4, 4, 6, 6, 6, 7, 7, 9, 9 }; int n = sizeof(sticks) / sizeof(sticks[0]); cout<<"The total number of squares or rectangles that can be created is "; cout<<countRecSqr(sticks, n); return 0; }
Output
The total number of squares or rectangles that can be created is 3