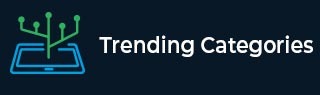
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
PHP Expressions
Introduction
Almost everything in a PHP script is an expression. Anything that has a value is an expression. In a typical assignment statement ($x=100), a literal value, a function or operands processed by operators is an expression, anything that appears to the right of assignment operator (=)
Syntax
$x=100; //100 is an expression $a=$b+$c; //b+$c is an expression $c=add($a,$b); //add($a,$b) is an expresson $val=sqrt(100); //sqrt(100) is an expression $var=$x!=$y; //$x!=$y is an expression
expression with ++ and -- operators
These operators are called increment and decrement operators respectively. They are unary operators, needing just one operand and can be used in prefix or postfix manner, although with different effect on value of expression
Both prefix and postfix ++ operators increment value of operand by 1 (whereas -- operator decrements by 1). However, when used in assignment expression, prefix makesincremnt/decrement first and then followed by assignment. In case of postfix, assignment is done before increment/decrement
Uses postfix ++ operator
Example
<?php $x=10; $y=$x++; //equivalent to $y=$x followed by $x=$x+1 echo "x = $x y = $y"; ?>
Output
This produces following result
x = 11 y = 10
Whereas following example uses prefix increment operator in assignment
Example
<?php $x=10; $y=++$x;; //equivalent to $x=$x+1 followed by $y=$x echo "x = $x y = $y"; ?>
Output
This produces following result
x = 11 y = 11
Expression with Ternary conditional operator
Ternary operator has three operands. First one is a logical expression. If it is TRU, second operand expression is evaluated otherwise third one is evaluated
Example
<?php $marks=60; $result= $marks<50 ? "fail" : "pass"; echo $result; ?>
Output
Following result will be displayed
pass