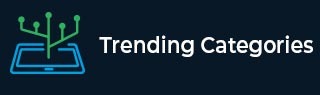
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Pairs of Songs With Total Durations Divisible by 60 in Python
Suppose we have a list of songs, the i-th song has a duration of time[i] seconds. We have to find the number of pairs of songs for which their total time in seconds is divisible by 60.
So if the time array is like [30, 20, 150, 100, 40], then the answer will be 3. Three pairs will be (3, 150), (20, 100), (20, 40) for all cases the total duration is divisible by 60.
To solve this, we will follow these steps −
- Take a map rem to store remainders. Set ans := 0
- for all elements i in time −
- if i is divisible by 0 and 0 in rem, then ans := ans + rem[0]
- o otherwise when 60 – (i mod 60) in rem, then ans := ans + rem[60 – (i mod 60)]
- if i mod 60 in rem, then rem[i mod 60] := rem[i mod 60] + 1
- otherwise rem[i mod 60] := 1
- return the ans
Example
Let us see the following implementation to get better understanding −
class Solution(object): def numPairsDivisibleBy60(self, time): ans = 0 remainder = {} for i in time: if i % 60 == 0 and 0 in remainder: ans += remainder[0] elif 60 - (i%60) in remainder: ans += remainder[60 - (i%60)] if i % 60 in remainder: remainder[i%60]+=1 else: remainder[i%60]=1 return ans ob1 = Solution() print(ob1.numPairsDivisibleBy60([30,20,150,100,40]))
Input
[30,20,150,100,40]
Output
3
Advertisements