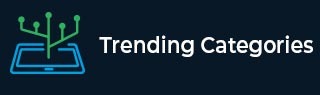
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Ordering string in an array according to a number in the string JavaScript
We have an array of strings each of which contain one or more numbers like this −
const arr = ['di5aster', 'ca1amity', 'cod3', 'ho2me3', 'ca11ing'];
We are required to write a sorting function that sorts this array in ascending order of the numbers present in the strings. The correct order will be −
const output = [ 'ca1amity', 'cod3', 'di5aster', 'ca11ing', 'ho2me3' ];
Therefore, let’s write the code for this problem −
Example
const arr = ['di5aster', 'ca1amity', 'cod3', 'ho2me3', 'ca11ing']; const filterNumber = str => { return +str .split("") .filter(el => el.charCodeAt() >= 48 && el.charCodeAt() <= 57) .join(""); }; const sorter = (a, b) => { return filterNumber(a) - filterNumber(b); }; arr.sort(sorter); console.log(arr);
Output
The output in the console will be −
[ 'ca1amity', 'cod3', 'di5aster', 'ca11ing', 'ho2me3' ]
Advertisements