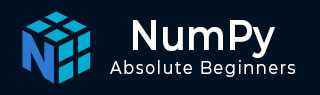
- NumPy Tutorial
- NumPy - Home
- NumPy - Introduction
- NumPy - Environment
- NumPy - Ndarray Object
- NumPy - Data Types
- NumPy - Array Attributes
- NumPy - Array Creation Routines
- NumPy - Array from Existing Data
- Array From Numerical Ranges
- NumPy - Indexing & Slicing
- NumPy - Advanced Indexing
- NumPy - Broadcasting
- NumPy - Iterating Over Array
- NumPy - Array Manipulation
- NumPy - Binary Operators
- NumPy - String Functions
- NumPy - Mathematical Functions
- NumPy - Arithmetic Operations
- NumPy - Statistical Functions
- Sort, Search & Counting Functions
- NumPy - Byte Swapping
- NumPy - Copies & Views
- NumPy - Matrix Library
- NumPy - Linear Algebra
- NumPy - Matplotlib
- NumPy - Histogram Using Matplotlib
- NumPy - I/O with NumPy
- NumPy Useful Resources
- NumPy Compiler
- NumPy - Quick Guide
- NumPy - Useful Resources
- NumPy - Discussion
numpy.append
This function adds values at the end of an input array. The append operation is not inplace, a new array is allocated. Also the dimensions of the input arrays must match otherwise ValueError will be generated.
The function takes the following parameters.
numpy.append(arr, values, axis)
Where,
Sr.No. | Parameter & Description |
---|---|
1 | arr Input array |
2 | values To be appended to arr. It must be of the same shape as of arr (excluding axis of appending) |
3 | axis The axis along which append operation is to be done. If not given, both parameters are flattened |
Example
import numpy as np a = np.array([[1,2,3],[4,5,6]]) print 'First array:' print a print '\n' print 'Append elements to array:' print np.append(a, [7,8,9]) print '\n' print 'Append elements along axis 0:' print np.append(a, [[7,8,9]],axis = 0) print '\n' print 'Append elements along axis 1:' print np.append(a, [[5,5,5],[7,8,9]],axis = 1)
Its output would be as follows −
First array: [[1 2 3] [4 5 6]] Append elements to array: [1 2 3 4 5 6 7 8 9] Append elements along axis 0: [[1 2 3] [4 5 6] [7 8 9]] Append elements along axis 1: [[1 2 3 5 5 5] [4 5 6 7 8 9]]
numpy_array_manipulation.htm
Advertisements