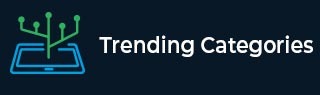
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Numeric for in Lua Programming
In Lua, there are two types of for loops − the numeric for and the generic for.
Syntax
The numeric for uses the following syntax −
for var=exp1,exp2,exp3 do something end
It should be noted that we can write exp1, exp2, exp3 at the same time or we can omit one of them, and the numeric loop will not result in a compile error, though its functionality will change.
Example
Let’s consider a simple variation of a numeric for loop, where we will try to print numbers from 1 to 10.
Consider the example shown below −
for i = 1, 10 do print(i) end
Output
1 2 3 4 5 6 7 8 9 10
Okay, that was simple! How about printing the numbers in a backward order? In that case, we need the exp3 that was mentioned in the syntax of the numeric for loop.
Example
Consider the example shown below which will print the numbers from 10 to 1.
for i = 10, 1, -1 do print(i) end
Output
10 9 8 7 6 5 4 3 2 1
Now, let’s explore a more common and useful case, where we want to iterate over an array in Lua, and print the values that are present inside the array.
Example
Consider the example shown below −
names = {'John', 'Joe', 'Steve'} for i = 1, 3 do print(names[i]) end
Output
John Joe Steve