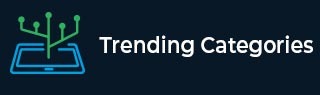
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Numbers With Same Consecutive Differences in C++
Suppose we have to find all non-negative integers of length N such that the absolute difference between every two consecutive digits is K. We have to keep in mind that every number in the answer must not have leading zeros except for the number 0 itself. We may return the answer in any order. So if N = 3 and K = 7, then output will be [181,292,707,818,929], Here we can see 070 is not a valid number, because it has one leading zero.
To solve this, we will follow these steps −
Create one matrix called dp, and its size will be n + 1, fill 1 to 9 into dp[1]
for i in range 1 to N – 1
Define a set called visited
for j in range 0 to size of dp[i]
x := dp[i, j]
lastNum := last digit of x
digit := lastNum + k
if digit is in range 0 to 9, and (x*10 + digit) is not visited, then
insert (10*x + digit) into dp[i + 1]
insert 10*x + digit into visited array
digit := lastNum – K
if digit is in range 0 to 9, and (x*10 + digit) is not visited, then
insert (10*x + digit) into dp[i + 1]
insert 10*x + digit into visited array
if N is 1, then insert 0 into dp[N]
return dp[N]
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; void print_vector(vector<int> v){ cout << "["; for(int i = 0; i<v.size(); i++){ cout << v[i] << ", "; } cout << "]"<<endl; } class Solution { public: vector<int> numsSameConsecDiff(int N, int K) { vector <int> dp[N + 1]; for(int i = 1; i <= 9; i++){ dp[1].push_back(i); } for(int i = 1; i < N; i++){ set <int> visited; for(int j = 0; j < dp[i].size(); j++){ int x = dp[i][j]; int lastNum = x % 10; int digit = lastNum + K; if(digit >= 0 && digit <= 9 && !visited.count(x * 10 + digit)){ dp[i + 1].push_back(x * 10 + digit); visited.insert(x * 10 + digit); } digit = lastNum - K; if(digit >= 0 && digit <= 9 && !visited.count(x * 10 + digit)){ dp[i + 1].push_back(x * 10 + digit); visited.insert(x * 10 + digit); } } } if(N == 1){ dp[N].push_back(0); } return dp[N]; } }; main(){ Solution ob; print_vector(ob.numsSameConsecDiff(3,7)); }
Input
3 7
Output
[181,292,707,818,929]