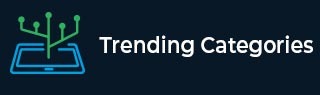
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Number of Recent Calls in Python
Suppose we want to write a class called RecentCounter to count recent requests. This class has only one method: ping(t), where t is representing some time in milliseconds. This will return the number of pings that have been made from 3000 milliseconds ago until now. Any ping with time in [t - 3000, t] will count, including the current ping. And it is guaranteed that every call to ping uses a strictly larger value of t than before.
So, if the input is like Call ping four times ping(1), ping(100), ping(3001), ping(3002), then the output will be 1,2,3,3 respectively.
To solve this, we will follow these steps −
- Initialize the class by making one queue, initially this is empty
- Define a function ping(). This will take t
- while size of queue is not 0 and t - queue[0] > 3000, do
- delete first element from queue
- insert t at the end of queue
- return size of queue
Let us see the following implementation to get better understanding −
Example
class RecentCounter: def __init__(self): self.queue = [] def ping(self, t): while len(self.queue) and t - self.queue[0] > 3000: self.queue.pop(0) self.queue.append(t) return len(self.queue) ob = RecentCounter() print(ob.ping(1)) print(ob.ping(100)) print(ob.ping(3001)) print(ob.ping(3002))
Input
ob.ping(1) ob.ping(100) ob.ping(3001) ob.ping(3002)
Output
1 2 3 3
Advertisements