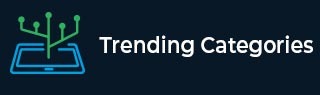
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Number of NGEs to the right in C++
You are given an array and the index of the target element. We have to count the number of elements greater than the given element to its right. Let's see an example.
Input
arr = [2, 3, 5, 1, 4, 2, 6] index = 3
Output
3
The target index element is 1. There are three elements i.e..., 4, 2, 6 that are greater than 1 on its right side.
Algorithm
- Initialise the array and index of target element.
- If the index is greater than or equal to the length of the array, then return -1.
- Write a loop that iterates from the next element of the given index.
- Increment the count if the element is greater than the target element.
- Return the count.
Implementation
Following is the implementation of the above algorithm in C++
#include <bits/stdc++.h> using namespace std; int getNextGreaterElementsCount(int arr[], int n, int index) { if (index >= n) { return -1; } int count = 0; for (int i = index + 1; i < n; i++) { if (arr[index] < arr[i]) { count += 1; } } return count; } int main() { int arr[] = { 1, 2, 3, 4, 5, 6, 7, 8 }; int n = 8, index = 1; cout << getNextGreaterElementsCount(arr, n, index) << endl; return 0; }
Output
If you run the above code, then you will get the following result.
6
Advertisements