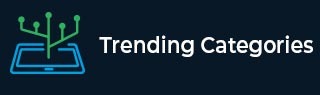
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Number of flips to make binary string alternate - Set 1 in C++
Let's say you have given a binary string "10011". To make an alternate binary string, we need to flip a minimum of 2 characters as "10101".
There are two possibilities for the alternate string. It will start with 0 or 1. We will check for two alternates and count the number of flips required for both.
And then return the minimum of both. Let's see an example.
Input
binary = "10011"
Output
2
If we start the string with 0, then we have to flip 3 times. And if we start the string with 1, then we have to flip 2 times. The minimum is 2.
Algorithm
- Initialise the binary string.
- Count the flips required to make string alternate starting with 1.
- Similarly count the flips required to make string alternate starting with 0.
- Find the minimum from the above two.
- Print the menimum.
Implementation
Following is the implementation of the above algorithm in C++
#include <bits/stdc++.h> using namespace std; char flip(char binaryDigit) { return binaryDigit == '0' ? '1' : '0'; } int getFlipCountToAlternateString(string binary, char expected) { int flipCount = 0; for (int i = 0; i < binary.length(); i++) { if (binary[i] != expected) { flipCount++; } expected = flip(expected); } return flipCount; } int main() { string binary = "10011"; cout << min(getFlipCountToAlternateString(binary, '0'), getFlipCountToAlternateString(binary, '1')) << endl; return 0; }
Output
If you run the above code, then you will get the following result.
2
Advertisements