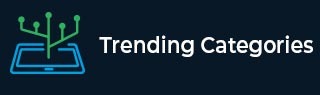
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Number of arrays of size N whose elements are positive integers and sum is K in C++
We are given two numbers n and k. We need to find the count of arrays that can be formed using the n numbers whose sum is k.
The number of arrays of size N with sum K is $\dbinom{k - 1}{n - 1}$.
This a straightforward formula to find the number arrays that can be formed using n elements whose sum k. Let's see an example.
Input
n = 1 k = 2
Output
1
The only array that can be formed is [2]
Input
n = 2 k = 4
Output
3
The arrays that can be formed are [1, 3], [2, 2], [3, 1].
Algorithm
- Initialise the numbers n and k.
- Write a function to calculate the factorial of a number.
- Now, write our main function to compute the binomial as seen above.
- Return the answer.
Implementation
Following is the implementation of the above algorithm in C++
#include <bits/stdc++.h> using namespace std; int factorial(int n) { int result = 1; for (int i = 2; i <= n; i++) { result *= i; } return result; } int getNumberOfArraysCount(int n, int k) { return factorial(n) / (factorial(k) * factorial(n - k)); } int main() { int N = 5, K = 8; cout << getNumberOfArraysCount(K - 1, N - 1) << endl; return 0; }
Output
If you run the above code, then you will get the following result.
35
Advertisements