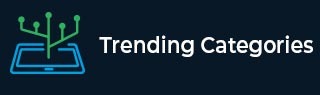
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Node.js – util.inherits() Method
The util.inherits() method basically inherits the methods from one construct to another. This prototype will be set to a new object to that from the superConstructor.
By doing this, we can mainly add some validations to the top of Object.setPrototypeOf(constructor.prototype, superConstructor.prototype).
Syntax
util.inherits(constructor, superConstructor)
Parameters
The parameters are described below -
- constructor − This is a function type input that holds the prototype for constructor the user wants to be inherited.
- superConstructor − This is the function that will be used for adding and validating the input validations.
Example 1
Create a file "inherits.js" and copy the following code snippet. After creating the file, use the command "node inherits.js" to run this code.
// util.inherit() example // Importing the util module const util = require('util'); const EventEmitter = require('events'); // Defining the constructor below function Stream() { EventEmitter.call(this); } // Inheriting the stream constructor util.inherits(Stream, EventEmitter); // Creating the prototype for the constructor with some data Stream.prototype.write = function(data) { this.emit('data', data); }; // Creating a new stream constructor const stream = new Stream(); // Checking if stream is instanceOf EventEmitter console.log(stream instanceof EventEmitter); // true console.log(Stream.super_ === EventEmitter); // true // Printing the data stream.on('data', (data) => { console.log(`Data Received: "${data}"`); }); // Passing the data in stream stream.write('Its working... Created the constructor prototype!');
Output
C:\home
ode>> node inherits.js true true Dta Received: "It's working... Created the constructor prototype!"
Example 2
Let’s have a look at one more example
// util.inherits() example // Importing the util & events module const util = require('util'); const { inspect } = require('util'); const emitEvent = require('events'); // Definging the class to emit stream data class streamData extends emitEvent { write(stream_data) { // This will emit the data stream this.emit('stream_data', stream_data); } } // Creating a new instance of the stream const manageStream = new streamData('default'); console.log("1.", inspect(manageStream, false, 0, true)) console.log("2.", streamData) manageStream.on('stream_data', (stream_data) => { // Prints the write statement after streaming console.log("3.", `Data Stream Received: "${stream_data}"`); }); // Write on console manageStream.write('Inheriting the constructor & checking from superConstructor');
Output
C:\home
ode>> node inherits.js 1. streamData { _events: [Object: null prototype] {}, _eventsCount: 0, _maxListeners: undefined, [Symbol(kCapture)]: false } 2. [class streamData extends EventEmitter] 3. Data Stream Received: "Inheriting the constructor & checking from superConstructor"
Advertisements